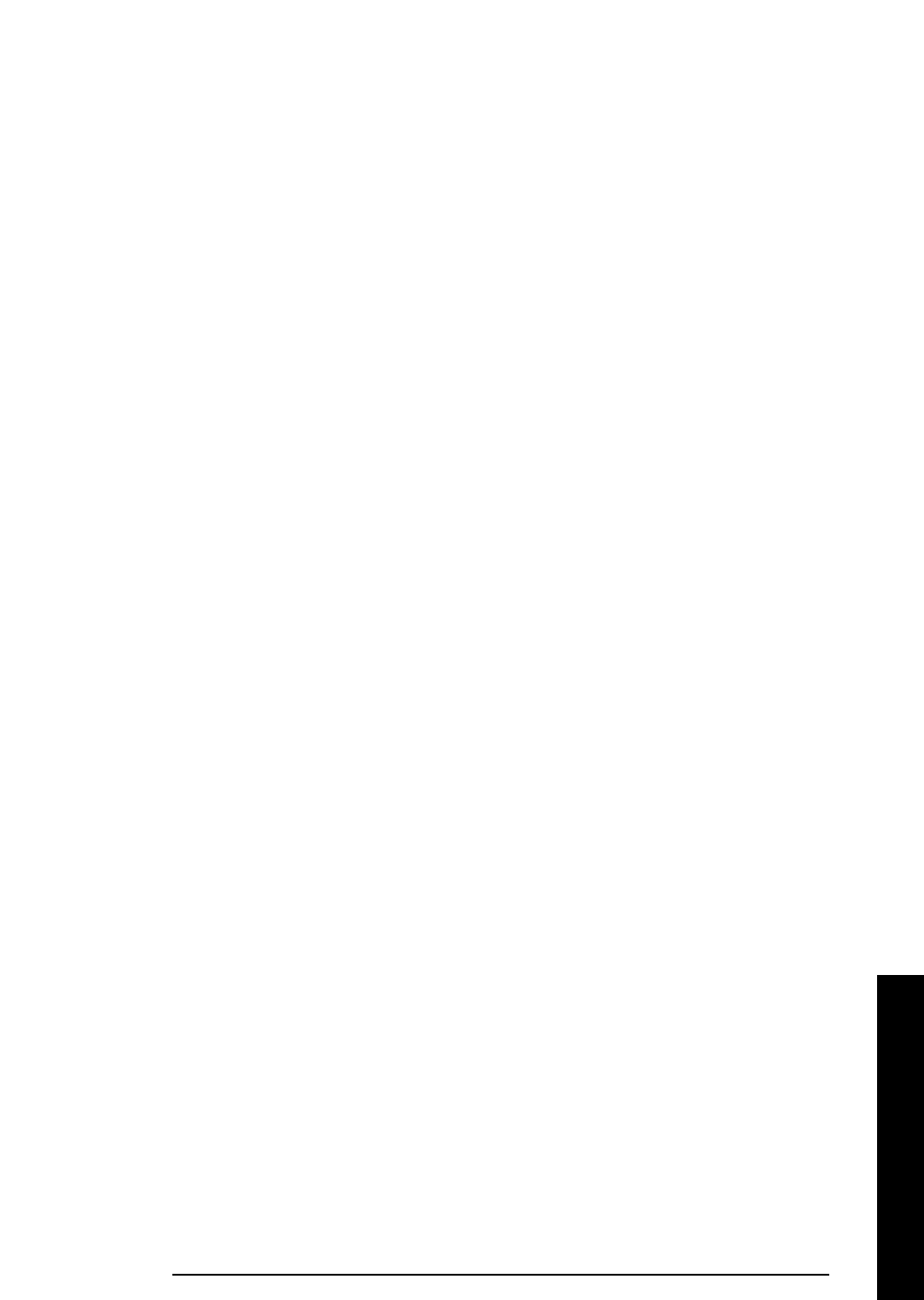
Chapter 6 549
Programming Fundamentals
Programming in C Using the VTL
Programming Fundamentals
Example Program
This example program queries a GPIB device for an identification
string and prints the results. Note that you must change the address.
/*idn.c - program filename */
#include "visa.h"
#include <stdio.h>
void main ()
{
/*Open session to GPIB device at address 18 */
ViOpenDefaultRM (&defaultRM);
ViOpen (defaultRM, GPIB0::18::INSTR", VI_NULL,
VI_NULL, &vi);
/*Initialize device */
viPrintf (vi, "*RST\n");
/*Send an *IDN? string to the device */
printf (vi, "*IDN?\n");
/*Read results */
viScanf (vi, "%t", &buf);
/*Print results */
printf ("Instrument identification string: %s\n", buf);
/* Close sessions */
viClose (vi);
viClose (defaultRM);
}
Including the VISA Declarations File
For C and C++ programs, you must include the visa.h header file at
the beginning of every file that contains VTL function calls:
#include "visa.h"
This header file contains the VISA function prototypes and the
definitions for all VISA constants and error codes. The visa.h header
file includes the visatype.h header file.
The visatype.h header file defines most of the VISA types. The VISA
types are used throughout VTL to specify data types used in the
functions. For example, the viOpenDefaultRM function requires a
pointer to a parameter of type ViSession.IfyoufindViSession in the
visatype.h header file, you will find that ViSession is eventually
typedasanunsignedlong.
Opening a Session
A session is a channel of communication. Sessions must first be opened